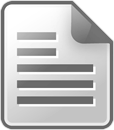
One of the things any aspiring security professional or network admin should know is - what is exactly a socket and what do they do?. This is tutorial is a different approach that I have been toying with on how to best explain to beginning IT or security students who are just beginning, but have no experience using "low-level" languages like C. One of my esteemed professor's once said: "God intended us to program in C" and I believe that today's programmers should begin with a language which will teach them responsibility. C is almost as close to the hardware you can go albeit learning assembly language and therefore the most basic understanding of what a socket is combined with a tidbit of knowledge in C.
What Is A Socket??
According to Wikipedia: A network socket is an endpoint of an inter-process communication flow across a computer network. Today, most communication between computers is based on the Internet Protocol; therefore most network sockets are Internet sockets.
A socket address is the combination of an IP address and a port number, much like one end of a telephone connection is the combination of a phone number and a particular extension. Based on this address, internet sockets deliver incoming data packets to the appropriate application process or thread.
OK so a socket is essentially, an interface address bound to a port and therefore a socket is the combination of the two!
What Does A Socket Mean To Me??
Well if a socket is an IP and a port - if you see one on your system, that means that you have an application running that is able to send or receive connections from the network. If your auditing your system(s), and there is a previously unknown application with network access - you should check it out!
Lets Create A TCP Echo Server and Client
While there are many tutorials on the Internet that beat this subject up until it is bloody, I want to work my way through it in layman's terms and explain how a security professional or IT technician can detect or monitor which sockets are open on their systems. In other words, your going to create a TCP Echo Server in C using sockets!
If you are in any modern Linux distribution, install an editor or IDE of your choice - I prefer Geany, but gedit would be okay. Also install gcc.
Create two files: server.c & client.c with the following code in each of them. I'll explain it as I go:
/* Almost always default includes for any C program */ #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> /* These three includes provide the functionality required by network sockets */ #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> /* This function is here just to print out any errors */ void error(const char *msg) { perror(msg); exit(1); } /* In C, each program has a main that accepts a number of arguments that are * passed in through stdin */ int main(int argc, char *argv[]) { return (0); }
So here is what you have done, you have added the necessary includes that sockets require in C. Think of these like includes in PHP or the imports in Java. The last two includes are the most important for sockets; sys/socket.h and netinet/in.h
Following that you have created a function that will print out any errors should they occur; these errors could mean that a socket is previously in use or you don't have permission to create a socket for example. After that there is a main function that accepts two arguments: int argc and char *argv[ ]. Every C program has a main function which contains the code that will be executed when the binary is ran. It returns a 0 before it exits, and its two parameters are:
- argc is an integer, which is the number of arguments when the binary was executed - the command or binary is included in the count.
- argv[] is an array of the arguments being given to the program on execution
Inside the main, at the top of the function add the following variable declarations:
/* integers that will be our socket descriptors */ int sockfd, newsockfd; /* integer that will be our port number */ int portno; /* Size_t type that will contain client length */ socklen_t clilen; /* Buffer that will contain our client/server data */ char buffer[256]; /* Socket address structures for the server and client sockets */ struct sockaddr_in serv_addr, cli_addr; /* Integer that will store any bytes transferred over the network */ int n;
Each function is fairly self-explanatory, but take notice of the buffer and sockaddr variables. Below the variable declarations, add the following if statement - it checks to see if you the user has given the application a port number when the application was executed.
/* Check number of arguments, if there is less than two then exit * Note: the binary counts as one. IE: ./server (1) port (2) */ if (argc < 2) { fprintf(stderr, "ERROR, no port provided\n"); exit(1); }
After the above code, add the following snippet of code that will create a socket. If you recall Linux's internals, everything in Linux uses file descriptors - sockets use them too! The socket function call will create a TCP socket with no options - this is set using the AF_INET parameter which states that the socket will be using the TCP/IP family and SOCK_STREAM which specifies that the socket will be TCP. Then following that there is an if statement that checks for an error opening the socket.
/* Create a TCP/IP TCP socket and assign it to sockfd using socket() */ sockfd = socket(AF_INET, SOCK_STREAM, 0); /* If socket returns an error, print one out */ if (sockfd < 0) { error("ERROR opening socket"); }
Next, we setup and configure the server's socket_address structure. Essentially, this code specifies that the socket will use the port number argument supplied by the user, for it to use any network interface and using the bind function call - bind the socket address structure to the file descriptor. Add the following code again below the previous snippet.
/* Clear and set the serv_addr structure to all zeros in memory */ bzero((char *) &serv_addr, sizeof(serv_addr)); portno = atoi(argv[1]); // Set the port number to this /* Setup server structure so the socket can be bound to the socket descriptor */ serv_addr.sin_family = AF_INET; serv_addr.sin_addr.s_addr = INADDR_ANY; serv_addr.sin_port = htons(portno); // portno must be converted to network byte order /* Bind the server file descriptor to the server socket address */ if (bind(sockfd, (struct sockaddr *) &serv_addr, sizeof(serv_addr)) < 0) { error("ERROR on binding"); }
The following chunk of code sets the socket to listen for up to five connections, and to accept any new connections and assign them to sockfd.
/* Listen for up to five incoming connections */ listen(sockfd, 5); clilen = sizeof(cli_addr); /* Accept new client connections */ newsockfd = accept(sockfd, (struct sockaddr *) &cli_addr, &clilen); /* Print error if there is an error on accepting a new client connection */ if (newsockfd < 0) { error("ERROR on accept"); }
Between the above code and the return 0 statement, add the following code that zeros the receiving buffer, reads any incoming data to the buffer, sends the string "I got your message" back to the client using the write function and closes both sockets.
/* Zero the buffer to make sure there is no extra garbage * that was previously in memory */ bzero(buffer, 256); /* Read from the buffer 255 bytes (from the incoming connection) * Note: 255 to prevent an overflow ;) */ n = read(newsockfd, buffer, 255); /* If no bytes were read - print an error */ if (n < 0) { error("ERROR reading from socket"); printf("Here is the message: %s\n", buffer); } printf("We received: %s\n", buffer); /* Now write "I got your message" back to the client (this is 18 bytes) */ n = write(newsockfd, "I got your message", 18); /* If no bytes were not sent - print an error */ if (n < 0) { error("ERROR writing to socket"); } /* Remember to close the socket descriptors for clean programming */ close(newsockfd); close(sockfd);
Now that you have all of the essential code - you will need a client! We could duplicate the code and create one OR we can cheat and use Netcat or NC to generate text for the server. To do this see if you have nc installed by typing nc in the terminal and press Enter. I won't tell you what netcat is great for (its like a networking swiss army knife or backdoor), but if your bored look at the man page for it.
Now we will compile the application with gcc, execute it and send it a test message:
In one terminal: gcc server.c -o server sudo ./server 6000 In a second terminal: echo "Test message" | nc 127.0.0.1 6000 In both terminals you should see a message in the output.
Now that you have verified your server works correctly, run it again, but do not send it a message. Lets change gears and put on the security hat - how can you tell that there are open sockets on the system? The answer is, you can use lsof , ps and netstat. The netstat tool provides visibility into the GNU/Linux networking subsystem. With netstat, you can view currently active connections (on a per-protocol basis), view connections in a particular state (such as server sockets in the listening state), and many others. Here are some of the options that netstat provides and the features they enable.
View all TCP sockets currently active $ netstat --tcp View all UDP sockets $ netstat --udp View all TCP sockets in the listening state $ netstat --listening View the multicast group membership information $ netstat --groups Display the list of masqueraded connections $ netstat --masquerade View statistics for each protocol $ netstat --statistics Alternatively - port 6000 is listed below: [rbrash@ord Desktop]$ netstat -ltn Active Internet connections (only servers) Proto Recv-Q Send-Q Local Address Foreign Address State tcp 0 0 0.0.0.0:49001 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:875 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:55085 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:111 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:6000 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:20048 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:22 0.0.0.0:* LISTEN tcp 0 0 127.0.0.1:631 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:36151 0.0.0.0:* LISTEN tcp 0 0 0.0.0.0:2049 0.0.0.0:* LISTEN tcp6 0 0 :::53578 :::* LISTEN tcp6 0 0 :::111 :::* LISTEN tcp6 0 0 :::20048 :::* LISTEN tcp6 0 0 :::22 :::* LISTEN tcp6 0 0 :::631 :::* LISTEN tcp6 0 0 :::33112 :::* LISTEN tcp6 0 0 :::2049 :::* LISTEN
One thing to note that running binaries can be running using a name different than that of the binary itself - lsof is one tool that can help you find them as well.
[rbrash@ord Desktop]$ sudo lsof -c server COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME server 8870 root cwd DIR 253,2 4096 24641550 /home/rbrash/Desktop server 8870 root rtd DIR 253,1 4096 2 / server 8870 root txt REG 253,2 9108 24641712 /home/rbrash/Desktop/server server 8870 root mem REG 253,1 158440 2231555 /usr/lib64/ld-2.15.so server 8870 root mem REG 253,1 2065544 2231556 /usr/lib64/libc-2.15.so server 8870 root 0u CHR 136,0 0t0 3 /dev/pts/0 server 8870 root 1u CHR 136,0 0t0 3 /dev/pts/0 server 8870 root 2u CHR 136,0 0t0 3 /dev/pts/0 server 8870 root 3u IPv4 291154 0t0 TCP *:x11 (LISTEN)
Conclusion
Hopefully by now - you have a better understanding of what sockets are and what you can do to find them running on your system - note that in linux is common for normal system processes to use sockets for communication!
I borrowed liberally from this tutorial, but if you want more information - here it is: http://www.linuxhowtos.org/C_C++/socket.htm
Comments
Explaining Sockets and Terrifying You With C | Pacific
Submitted by Josh (not verified) on
I actually have a tendency to go along with all the stuff that ended up being
composed within “Explaining Sockets and Terrifying You With C |
Pacific Simplicity”. Thanks for all of the tips.I appreciate it,Theron
Add new comment